Syllabus
Chapter 1: Preface
User Guide
Chapter 2: Introduction
Introduction to the course and a simple program, Basic commands, Program structure and syntax
Chapter 3: Problem Solving Using A Computer
Real life problems as math problems, Problem solving and algorithms without computers, Representing numbers on
a computer, Machine Language and How a computer works
Chapter 4: Variables and data types
Variables and data types, Assignment statements, Arithmatic expressions, Reassignment, Sequence generation and
accumulation, Blocks and scope
Chapter 5: Program-design example
Specification and construction of test cases, Translate manual algorithm to a program, Debugging
Chapter 6: Simplecpp graphics
Turtle, Co-ordinate system, Creating multiple turtles, Creating graphical objects, Commands
Chapter 7: Conditional execution
Basic if statement, Most general form of if, Most general form of conditions, A somewhat large program example,
Switch statement and logical data
Chapter 8: Loops
Loops, Mark averaging, The break and continue statements, The for statement, Euclid's algorithm for GCD,
Correctness proof for GCD
Chapter 9: Computing common mathematical functions
Taylor series, Numerical Integration, Bisection Method, Newton Raphson Method
Chapter 10: Loops in various applications
Brute force for GCD, Finding Pythagorean Triples, Modelling a system: bargaining, Simulating a water tank,
Arithmetic on very large numbers
Chapter 11: Functions
Functions basics, Examples, Reference parameters, Pointers, Graphics Objects
Chapter 12: Recursive Functions
Recursive objects, Tree drawing, How to think about recursion, Virahanka Numbers
Chapter 13: Program Organization and Functions
Splitting a program into files, Namespaces, How to use C++ without simplecpp
Chapter 14: Functions: Advanced Topics
Introduction and passing one function to another, Lambda expressions, Default values to parameters,
Function overloading
Chapter 15: Arrays
Arrays, Arrays of graphical objects , Arrays and function calls, Sorting an array
Chapter 16: More on Arrays
Textual data, Functions on character strings, Two dimensional arrays, Command Line Arguments
Chapter 17: Arrays and Recursion
Binary Search Introduction, Binary search analysis, Mergesort overview, Merge function
Chapter 18: Object-oriented Programming: Structures
Definition and instantiation, Operations on structures, Pointers, Introduction to Member functions,
Vectors from Physics
Chapter 19: Object Oriented Programming: Classes
Constructors, Operator overloading, Access control, Classes for graphics and input output
Chapter 20: Representing Variable length Entities
Heap memory basics, Pitfalls of using heap memory, Automating memory management, Implementing a class with
automated memory management, Using the implemented class
Chapter 21: The Standard Library
Class string, Class vector, Sorting vectors and arrays, Classes map and unordered_map, Iterators
Chapter 22: Data Structure based programming
Set and pair classes, Implementation of standard library data structures, Composing data structures, typedef
Chapter 23: Medium Size Programs
The new marks display program, Manual algorithm for new marks display, RSMTAB and rest of the program,
Sophisticated solutions to marks display
Chapter 24: A graphical editor and solver for circuits
Outline, Main program and organization, Mathematical representation of the circuit, Extensions
Chapter 25: Cosmological simulation
First order Euler method, Second order Euler method
Level
Undergraduate
Prerequisites
None
Category
Self-paced
Estimated Time
50 hours
Meet the instructor
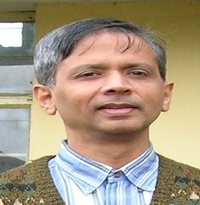
Abhiram G. Ranade
Abhiram G. Ranade is a professor of Computer Science and Engineering
at IIT Bombay. He obtained a B. Tech. degree in Electrical Engineering from IIT Bombay in 1981. In 1988 he
obtained a Ph.D. in Computer Science from Yale University, USA. He was an Assistant professor of Electrical
Engineering and Computer Science at the University of California, Berkeley, USA during 1988-94. Since 1995 he
has been a faculty member in IIT Bombay.
His research interests are Algorithms, Combinatorial Optimization, Scheduling in Transportation Systems, and Programming Education. He has won Excellence in Teaching Awards of IIT Bombay in 2006-7 and 2010-11.
His research interests are Algorithms, Combinatorial Optimization, Scheduling in Transportation Systems, and Programming Education. He has won Excellence in Teaching Awards of IIT Bombay in 2006-7 and 2010-11.